Learning Objectives
- Learn the different types of color blindness.
- Implement the Object-Oriented concept: class inheritance
- Implement the Object-Oriented concept: interfaces
Introduction
Delve into the world of color blindness, a condition that affects how individuals perceive and interact with the visual world. The core of this assignment revolves around creating statements explaining color blindness using Java. Students will utilize Object-Oriented Programming concepts such as class inheritance and interfaces to model different types of color blindness accurately.
Understanding Types of Color Blindness
We encourage students to explore the diverse spectrum of color blindness types. It's essential to have a comprehensive understanding of these conditions to effectively model them in code. You can find valuable resources with detailed information on different types of color blindness below but feel free to find other reputable sources on your own as well.
The Impact of Technology
Color blindness can pose unique challenges when it comes to technology. Many aspects of our digital world rely on color cues, from user interface to data visualizations. Students should consider the difficulties that individuals with color blindness might encounter when using technology.
Awareness
It is crucial to consider how color blindness can impact daily life. Including reading, interpreting data, and using technology. At the end of the assignment, you should reflect on questions like "How does color blindness affect people's ability to code?" and "What is something that can be improved upon in Computer Science classes to make it easier for people with color blindness to learn?" to appreciate the real-world implications of their work.
As you embark on this assignment, you will not only gain valuable technical skills in Java but also develop a deeper awareness of the challenges faced by those with color vision deficiencies. By the end of this assignment, you will be well-equipped to model and understand the diverse world of color blindness and its impact on technology and everyday life.
Object-Oriented Concepts
Class Inheritance
Class inheritance is a fundamental concept in Object-Oriented Programming. It allows a new class to reuse the code of an existing class. The new class is known as the subclass, and the existing class is called the based class. The subclass inherits all of the attributes and methods of the base class, but it can also have its own, unique attributes and methods as well. Inheriting the attributes and methods of the base class allows for the creation of hierarchical structure classes, with each class extending the functionality of the class before it.
Inheritance is also useful because it can make your code more reusable and it also reduces the amount of code duplication between two subclasses. There are two main types of inheritance in Object-Oriented Programming: single inheritance and multiple inheritance. In single inheritance, a subclass can only inherit from one base class. In multiple inheritance, a subclass inherits from multiple base classes. In this assignment, you should only worry about single inheritance.
In this assignment, we use class inheritance to create a hierarchy of color blindness types. This hierarchy allows us to model various forms of color blindness accurately, building upon a common foundation while customizing attributes and methods for each specific type.
Why is Inheritance important for this assignment?
- Code Reusability: By using class inheritance, we can reuse the code and attributes from a base class for various types of disabilities. This avoids redundancy, making the code more efficient and easier to maintain.
- Hierarchical Structure: Inheritance enables the creation of a hierarchical structure. Each color blindness type inherits characteristics from the base class and extends them to represent its unique features. This ensures a logical and structured representation of the different conditions.
- Uniform Interface: Inheritance enforces a consistent interface for the color blindness type. The methods and attributes inherited from the base class maintain a common structure, making it easier for developers to work with and understand the various type.
- Scalability: If we wanted to add more disabilities or make modification, inheritance simplifies the process. We can create new subclasses without altering the base class, which is critical for maintaining the integrity of the codebase.
At the end of this assignment, please answer the following questions and turn it in with your project.
- What is the purpose of class inheritance in this assignment?
- How does inheritance enhance code reusability and maintainability in the context of this assignment?
- What are the advantages of maintaining a hierarchical structure of classes for the various color blindness types?
Interfaces
Interfaces are also a fundamental part of Object-Oriented Programming. In this assignment, you use interfaces to define a contract that specifies what methods must be implemented by classes that implement the interface. Specifically, you have AssistiveTechnology, AccessibleDesign, and EnvironmentalAssistance.
Wht are Interfaces Important for This Assignment?
- Forcing Method Implementation: Interfaces ensure that any class implementing them must provide specific methods. In this assignment, these methods define behaviors associated with recommendations for color blindness assistance, making sure that they are consistent across all classes.
- Achieving Multiple Inheritance-like Behavior: Java supports multiple interfaces, allowing a class to implement more than one interface. In the context of your assignment, this is crucial because it enables classes to inherit and provide behaviors from multiple sources, without the complexity of multiple inheritance.
- Polymorphism and Flexibility: Interfaces support polymorphism, allowing objects of different classes to be treated as objects of a common interface. This flexibility is vital when you want to work with diverse color blindness types in a uniform way.
At the end of this assignment, please answer the following questions and turn it in with your project.
- What is the purpose of interfaces in this assignment?
- How do interfaces enforce consistency in method implementation across different classes in your assignment?
- Give an example of how interfaces enhance code modularity and maintainability in this assignment.
Assignment
In this assignment, your task is to model and simulate accessibility considerations for individuals with color blindness. You will work with a set of classes and interfaces designed to represent disabilities, specifically color blindness, and to explore the impact of various types of color blindness on individuals' daily lives. This includes simulating the adaptation and assistance required for navigating both physical and digital environments. Below is a walkthrough of the eight provided classes/interfaces to get a better understanding of the assignment. main been completed for you and the rest of them have skeleton code to help you get started.
1. Main
The entry point of your simulation. This class involves interacting with the user to create a persona, assign them a color blindness type, and generate a narrative that illustrates the persona's daily experiences and the assistance they receive. This class has been completed for you.
2. AssistiveTechnology
Dedicated to assistive devices and technologies, this interface requires you to outline a method for suggesting various technological aids that can improve the quality of life for individuals with disabilities.
TODO 1.1: Implement the AssistiveTechnology interface.
3. AccessibleDesign
This interface focuses on the digital aspect of accessibility. You will implement a method that recommends digital tools and software enhancements to aid users with disabilities in navigating digital content effectively.
TODO 1.2: Implement the AccessibleDesign interface.
4. EnvironmentalAssistance
This interface is intended for defining methods related to providing support in physical environments. Your task is to implement methods that suggest environmental modifications or aids to assist individuals with disabilities.
TODO 1.3: Implement the EnvironmentalAssistance interface.
5. Disability
A base class that models a generic disability with attributes for name and definition. You are provided with a constructor and getters that need to be implemented to initialize and return the respective attributes.
TODO 2.0: Implement the constructor and methods in the Disability class.
6. ColorBlindness
Extending Disability, this class represented a specific type of disability. It adds attributes to describe the impact of color blindness and its type. Implement the constructor, getters, and static methods to define and understand the effects of different types of color blindness.
TODO 3.0: Implement the constructor in the ColorBlindness class.
TODO 3.1: Implement the getImpactOnVision() method in the ColorBlindness class.
TODO 3.2: Implement the getType() method in the ColorBlindness class.
TODO 4.0: Give the definition of each type of color blindness in the ColorBlindness class.
TODO 4.1: Explain how each type of color blindness affects someone's vision in the ColorBlindness class.
7. AccessibilityRequirements
Aimed at implementing the three interfaces, this class should provide concrete recommendations for assistive technologies, digital tools, and environmental aids suitable for individuals with disabilities. Implement the methods to return useful suggestions and simulate a random assistance generator.
TODO 5.0: Make it so AccessibilityRequirements implements the three interfaces.
TODO 5.1: Implement the recommendedAssistiveTechnologies() method in the AccessibilityRequirements class.
TODO 5.2: Implement the digitalTools() method in the AccessibilityRequirements class.
TODO 5.3: Implement the environmentalAssistance() method in the AccessibilityRequirements class.
8. Human
Represents a person with a set of characteristics, including a type of color blindness. It uses AccessibilityRequirements to model necessary support. Your job is to complete the constructor and methods that assign a color blindness type, hobbies, and challenges, adding depth to the persona.
TODO 6.0: Implement Constructor in the Human class.
TODO 6.1: Implement the getName() method in the Human class.
TODO 6.2: Implement the getDisabilityType() method in the Human class.
TODO 6.3: Implement the setDisability() method in the Human class.
TODO 6.4: Implement the generateNarrative() method in the Human class.
TODO 6.5: Implement the setHobby() method in the Human class.
TODO 6.6: Implement the setChallenge() method in the Human class.
Sample Output
This output will not be about Color Blindness as it is your job to read and find the definitions, impacts on vision, and accessibility suggestions for each type of color blindness. Below is an example of how the output should be formatted and how in-depth you should be with each explanation.
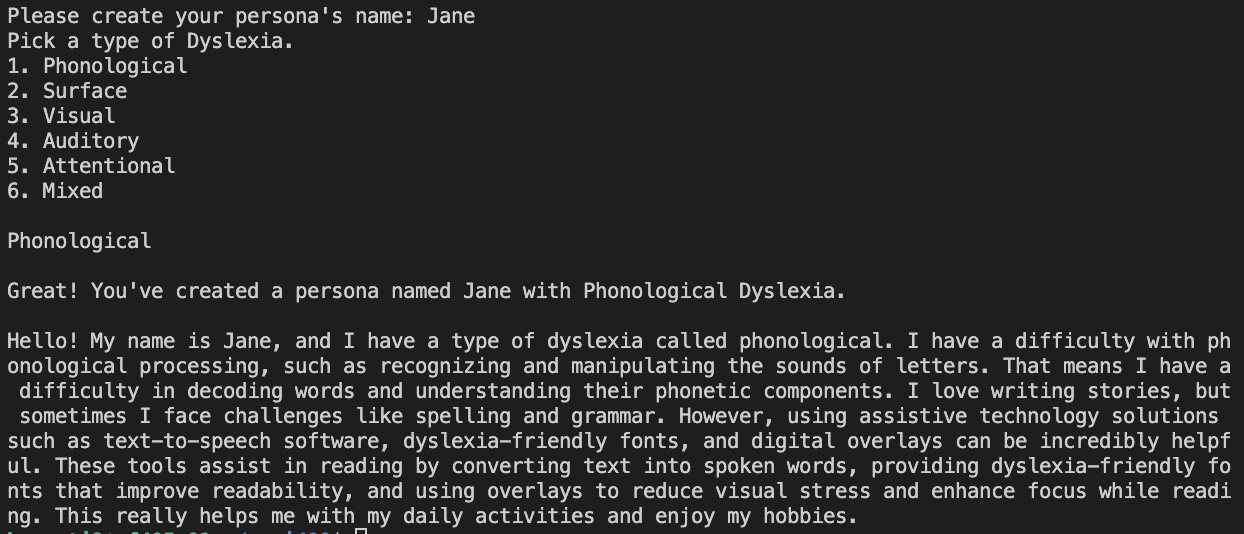
Project Plan and Reflection
You must write both a project plan named plan.txt and a reflection named reflection.txt. plan.txt must be completed before the start of the project and reflection.txt must be completed before submitting your project.
plan.txt should include the following information:
- A one paragraph summary of the program in your own words. What is being asked of you? What will you implement in this assignment?
- In 2-3 sentence, explain your thoughts on what you anticipate being the most challenging aspect(s) of the assignment.
- A proposed schedule for when you will work on this assignment with your partner and where you will meet.
- A list of at least 3 different resources you plan to use if you get stuck on something.
reflection.txt should include the following information:
- Declare/discuss any aspects of your code that are not working. What are your intuitions about why they are not working? Acknowledge and discuss any parts of the program that appear to be inefficient.
- What are some of the most important lessons you learned while working on this assignment? Why do you think so?
- What was the most challenging aspect of this assignment? Why?